Mastering the Python Built-in Debugger
As developers, we often find ourselves in situations where our code doesn't work as expected. In such scenarios, a debugger becomes an indispensable tool in our toolkit. A debugger allows us to inspect and analyze the execution of our code step by step, helping us identify issues, understand program flow, and ultimately, squash those pesky bugs. In this article, we'll delve into the world of Python's built-in debugger, exploring its fundamentals, key features, and real-world examples.
Understanding Debugging
Debugging is finding and fixing errors, or "bugs," in our code. These bugs can be logical errors that cause incorrect output or unexpected behavior. A debugger assists us in this process by providing tools to pause, inspect, and manipulate our code's execution in a controlled manner.
Tragically, the opposite of debugging is not commonly called "bugging", it's just referred to as plain old software development.
The Python Built-in Debugger: pdb
Python comes equipped with a built-in debugger called pdb
, short for "Python Debugger." The pdb
module provides an interactive debugging environment that allows us to step through our code line by line, inspect variables, and execute arbitrary code within the context of the program. Let's jump into some practical examples to understand how to use pdb
.
Mastery of Python's built-in debugger, pdb
, is a critical skill, especially in environments without robust IDEs. When faced with remote servers or command-line interfaces, pdb
becomes your go-to tool for pinpointing issues, regardless of the coding environment. This proficiency fosters self-reliance, deepens code understanding, and enhances collaboration among developers. By mastering pdb
, you're equipped with an adaptable debugging companion that transcends limitations and propels your coding expertise to new heights.
Debugger Commands
Let's go over the commands we have at our disposal before diving into a concrete example debugging workflow.
n
ornext
: Execute the current line and stop at the next line.s
orstep
: Step into a function call.c
orcontinue
: Continue execution until the next breakpoint is encountered.q
orquit
: Quit the debugger and terminate the program.p <expression>
: Print the value of an expression.l
orlist
: List the source code around the current line.h
orhelp
: Display a list of available debugger commands.
Basic Usage
To start debugging a script, simply add the following line at the point in your code where you want the debugger to kick in:
import pdb; pdb.set_trace()
This line will pause the execution of the script and launch the debugger. Let's consider a simple script to calculate the factorial of a number:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
result = factorial(5)
print("Factorial:", result)
If we add the import pdb; pdb.set_trace()
line just before the result = factorial(5)
line, the debugger will pause execution at that point. Once the debugger is active, you can enter various commands to navigate and inspect the program state.
Example Walkthrough
Set the Breakpoint
First things first, insert import pdb; pdb.set_trace()
just before result = factorial(5)
.
Your script should look like this:
def factorial(n):
if n == 0:
return 1
else:
return n * factorial(n - 1)
import pdb; pdb.set_trace()
print("start debugging here")
result = factorial(5)
print("Factorial:", result)
I have also added a new print statement to signify where we'll start the debugger, just for clarity.
Initiate Debugging
Execute the script, and watch the magic happen. The execution will pause, and you'll encounter the debugger's interactive prompt:
> /path/to/your/script.py(5)<module>()
-> result = factorial(5)
Executing the Current Line
It's time to take action! Type n
(short for "next") and hit Enter. This command will execute the current line and halt at the next line:
> /path/to/your/script.py(6)<module>()
-> print("Factorial:", result)
Printing a Variable
Now, let's peer into the heart of the program. Type p n
and hit Enter to print the value of n
, which in this case is 5:
(Pdb) p n
*** NameError: name 'n' is not defined
Uh-oh! Looks like n
is not available at the current execution line, which makes sense as we haven't entered our function body yet. Let's keep on trucking!
Stepping into a Function
Next, we'll delve into the depths of the factorial
function. Type s
(for "step") and press Enter. This command steps into the function:
--Call--
> /path/to/your/script.py(2)factorial()
-> def factorial(n):
Now we can try printing n
again.
(Pdb) p n
5
Woo!
Viewing Source Code
Curious about the surroundings? Type l
to view the source code around the current line. You'll be able to see the entire function definition:
1 def factorial(n):
2 if n == 0:
3 return 1
4 else:
5 return n * factorial(n - 1)
Executing within the Function
To move forward, type n
again. This will execute the current line within the function and pause at the next line:
> /path/to/your/script.py(3)factorial()
-> if n == 0:
Revisiting the Variable
Ready to observe the change? Type p n
once more to print the updated value of n
, which is now 4:
(Pdb) p n
4
Keep Moving Forward
Continue your journey by using n
, s
, and other commands to navigate through the code. Traverse through the loops and logic until you reach the script's end.
Farewell
Once you've unraveled the mysteries of your code, type q
to bid adieu to the debugger. It's time to put your newfound insights into action!
But wait, there's more!
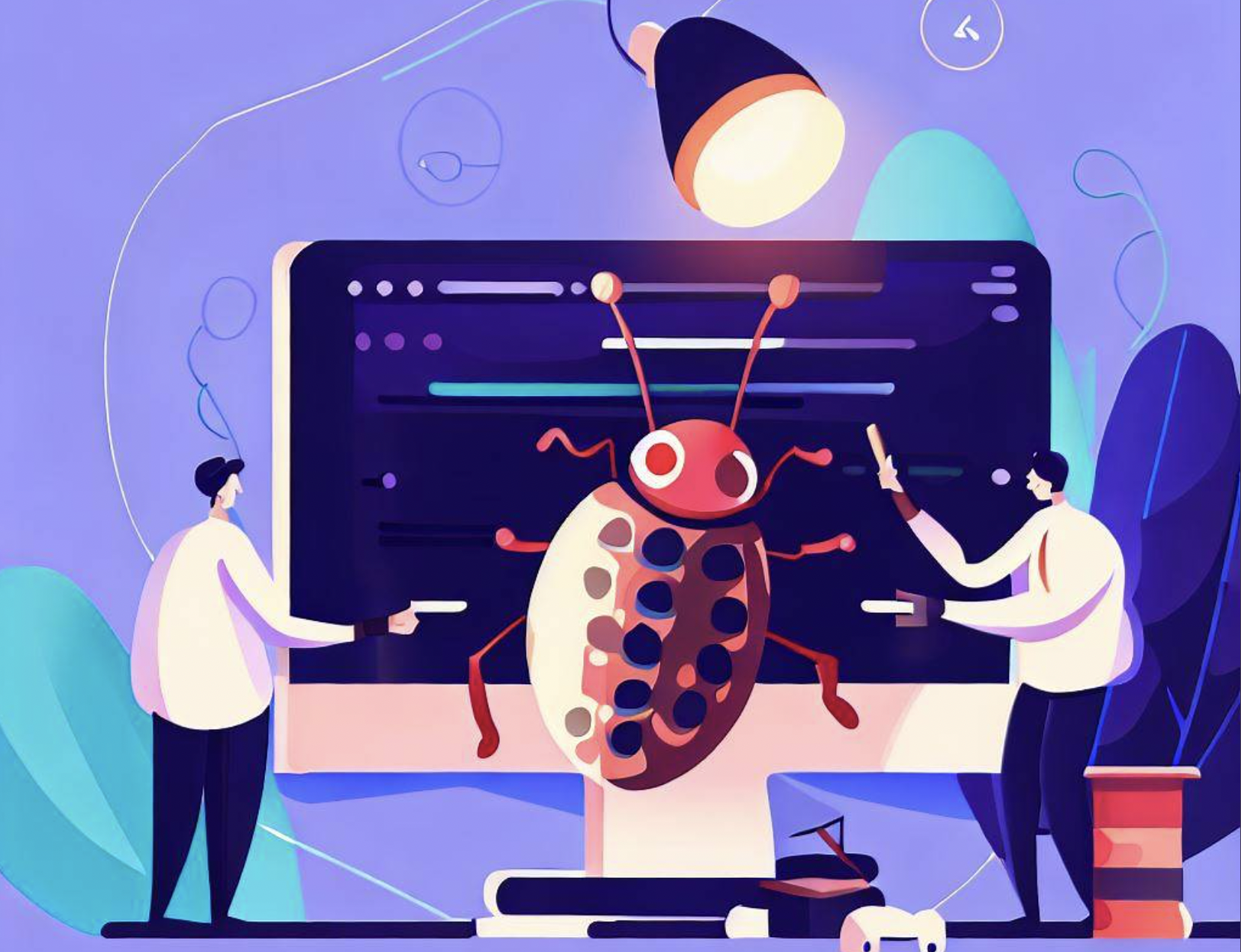
One of the most remarkable aspects of the pdb
debugger is its ability to transform your debugging experience into a full-fledged Python shell. This means that you can not only inspect variables and their values, but you can also execute arbitrary Python code within the context of your program. This feature elevates pdb
from a simple line-by-line debugger to a powerful exploration and experimentation tool.
Entering the Debugging Shell
Once you've triggered the debugger by adding import pdb; pdb.set_trace()
to your code, you're granted access to a debugging shell that lets you interact with your program. This shell provides you with the capability to explore variables, test hypotheses, and gain insights into your code's behavior.
Exploring Variables
You can use the pdb
shell to inspect the values of variables at any point during program execution. Let's go through some examples:
Print Variable Value
As we've seen before, suppose you have a variable counter, and you want to see its value:
(Pdb) p counter
42
Inspect Data Structures
If your script involves complex data structures, like a list named my_list
, you can examine its contents:
(Pdb) p my_list
[10, 20, 30, 40]
Access Object Attributes
If you have an object person
with attributes name
and age
, you can access them using dot notation:
(Pdb) p person.name
'Alice'
Executing Code
Now for something really cool. You can also execute code directly in the pdb
shell to experiment and understand behavior. Here's how:
Calculate Intermediate Value
Suppose you're debugging a function that involves some calculations. You can calculate intermediate values on the fly:
(Pdb) sum([1, 2, 3])
6
Modify Variables
You can modify variables to see how it affects program behavior. For example, you can update a variable x
:
(Pdb) x = 100
(Pdb) p x
100
Call Functions: You can call functions within the pdb
shell to test hypotheses or analyze behavior:
(Pdb) def double(num):
... return num * 2
...
(Pdb) double(7)
14
Example Demonstration
Consider debugging a function calculate_average
that calculates the average of a list of numbers. You've set a breakpoint using pdb
and entered the debugging shell:
def calculate_average(numbers):
total = sum(numbers)
avg = total / len(numbers)
return avg
numbers = [10, 20, 30, 40, 50]
import pdb; pdb.set_trace()
result = calculate_average(numbers)
print("Average:", result)
Once the debugger is active, you can interact with the variables and test hypotheses:
Inspect Numbers List
To view the list of numbers:
(Pdb) p numbers
[10, 20, 30, 40, 50]
Calculate Sum
Calculate the sum of the numbers:
(Pdb) sum(numbers)
150
Modify and Test
Modify the numbers
list and calculate the average again:
(Pdb) numbers.append(60)
(Pdb) p numbers
[10, 20, 30, 40, 50, 60]
(Pdb) result = calculate_average(numbers)
(Pdb) p result
35.0
Conclusion
The Python built-in debugger, pdb
, is an essential tool for any developer's debugging arsenal. With its interactive features and powerful commands, you can dive deep into your code, inspect variables, and understand program flow like never before. By practicing the techniques outlined in this article, you'll be better equipped to tackle even the most elusive bugs in your Python projects. Happy debugging!
Member discussion